- The Developer Bootcamp
- Posts
- Issue 1
Issue 1
require Vs import in JavaScript
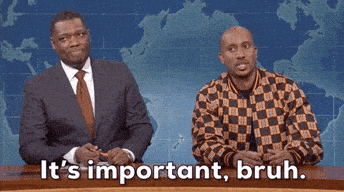
Today’s Issue: require vs import in JavaScript, cool things, and what I’m watching this weekend
Welcome to #1.
First Up
I remember when I started coding I would see some js files using require() to import modules and other files using import. This confused the heck out of me as I didn’t understand why there was inconsistency across projects. Here’s a quick rundown…
CommonJS (require)
CommonJS is a set of standards used in server-side JavaScript, typically Node.js environments
Modules load synchronously, which means the script execution is blocked until the module is loaded. This can slow things down and cause bottlenecks leading to bad performance if not handled correctly.
When using CommonJS, you’d use module.exports to export functionality and require() to import it.
// In file multiply.js
module.exports = function multiply(x, y) {
return x * y;
}
// In file main.js
const multiply = require('./multiply.js');
console.log(multiply(5, 4)); // Output: 20
ES6 (import)
ES6, also known as ECMAScript, is a newer version of JavaScript introduced in 2015.
Modules load asynchronously using the import statement which means the script running can continue to run while the module is being loaded in. This prevents bottlenecking and boosts performance.
Imports are statically analyzed by linters and bundlers which allows for an efficiency called tree-shaking which basically means removing unused/dead code from the bundle that is being sent to the browser. This also boosts performance.
When using ECMAScript, you’d use export to export functionality and import to import it.
// In file multiply.js
export const multiply = (x, y) => x * y;
// In file main.js
import { multiply } from './multiply.js';
console.log(multiply(5, 4)) // Output: 20
Basically using ECMAScript’s import is more optimized and easier to read, that’s why it is now the standard across the industry. The only times you’ll use require is if you are working on a legacy code base that was created before 2015 or if you need to conditionally load modules.
Hope this was helpful in explaining the difference. Go here if you’d like to read the full article I wrote about this.
Things I Found Interesting
If you’d like to watch a quick 100-second video explaining JavaScript modules check out this video by Fireship!
For anyone interested in design, here’s an awesome Figma tutorial I found that’s FREE!
Also, I just finished reading a book called The Believer: A Year in the Fly Fishing Life by David Coggins. In the book, Coggins journeys to Norway, Scotland, Spain, Cuba, Argentina, and a few places in the US including Wyoming, Tennessee, and the Catskills all the while documenting his journey along the way. It’s a great read for anyone interested in the outdoors or just likes a good story.
Enjoy Your Weekend!
This weekend I’ll be relaxing watching UFC 305 and enjoying some cold snacks (beer)! We’ll see if Adesanya can retain his title 🙂
That’s all for now, have a great weekend, and happy coding!
Reply